Introduction to Python¶
Resources¶
Unix shell¶
Python for Science¶
More extensive set of notes focusing on scientific computation with Python - probably more useful as a reference. Overlpas with our first few lectures.
Overview¶
# packages, modules, imports, namespaces
import numpy as np
from scipy.misc import factorial
# function definition with default arguments
def poisson_pmf(k, mu=1):
"""Poisson PMF for value k with rate mu."""
return mu**k*np.exp(-mu)/factorial(k)
# Jupyter notebook "magic" function
# Sets up "inline" plotting
%matplotlib inline
# Importing the seaborn plotting library and setting defaults
import seaborn as sns
sns.set_context("notebook", font_scale=1.5)
# Variable assignment
n = np.arange(10) # [0, 1, 2, ..., 0]
# Note that poisson_pmf is vectorized
sns.barplot(n, poisson_pmf(n, 2))
# pass is a do-nothing statement -
# Used here to suppress printing of return value for sns.barplot()
pass
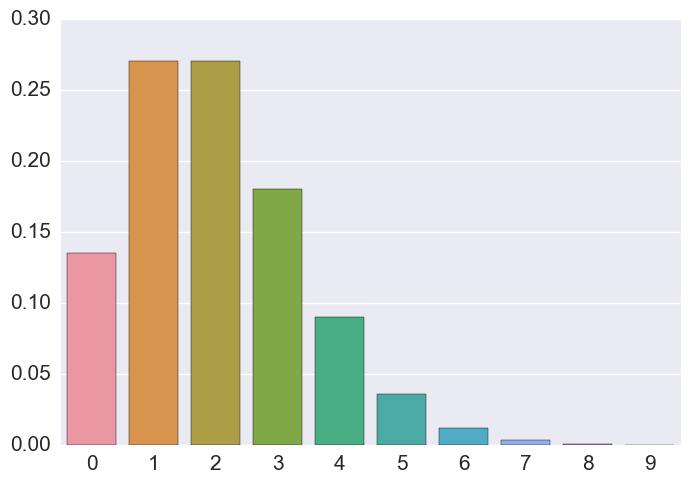
Types¶
# Boolean
True, False
(True, False)
# Integer
0, 1, 23, int(3.8)
(0, 1, 23, 3)
# Float
1.2, 3.14, float(2)
(1.2, 3.14, 2.0)
# Complex
1 + 2j, complex(23)
((1+2j), (23+0j))
# String
('abc', "abc",
"""abc
def
ghi""",
r'\t')
('abc', 'abc', 'abcndefnghi', '\t')
# None
None
type(3)
int
type(poisson_pmf)
function
Operators¶
2 * 3
6
2 ** 3
8
2 ^ 3 # danger, Will Robinson! ^ is bitwise exclusive-or, not exponentiation
1
7 /3
2.3333333333333335
7 // 3
2
2 < 3
True
7 % 3
1
1 == 1
True
1 != 2
True
a = [1,2,3]
b = a
c = [1,2,3]
b == a
True
b is a
True
c == a
True
c is a
False
np.array([1,2,3]) @ np.array([1,2,3])
14
True or False, True | False
(True, True)
True and False, False & True
(False, False)
2 << 4
32
fruits = ['apple', 'banana', 'cherry', 'durian', 'eggplant', 'fig']
'durian' in fruits
True
The operator module¶
Provides versions of operators as functions useful for the functional programming style.
import operator as op
op.mul(3, 4)
12
from functools import reduce
reduce(op.mul, [2,3,4,5], 1)
120
Names, assignment and identity¶
# Create some object (the list [1,2,3]) on the RHS and assign it to the name on the LHS
a = [1,2,3]
a
[1, 2, 3]
# Find the identity (address in memory in CPython) of the object named a
id(a)
4439213064
# Give the object named as a another name b
b = a
b
[1, 2, 3]
# b is just another name for the object also named a
# So the identity is the same
id(b)
4439213064
# Create a new object (the list [1,23]) and give it a name c
c = [1,2,3]
c
[1, 2, 3]
# The object named c has a different identity from the object with names a, b
id(c)
4684024264
a
[1, 2, 3]
b[0] = 99
a
[99, 2, 3]
c
[1, 2, 3]
Naming conventions¶
Collections¶
Tuples¶
course = ('STA-663', 2017, 'Spring', 50)
course[0]
'STA-663'
course[1]
2017
course[-1]
50
Tuple unpacking¶
name, year, semester, size = course
semester
'Spring'
name, *when, size = course
name
'STA-663'
size
50
when
[2017, 'Spring']
Named tuples¶
import collections
course = collections.namedtuple('course', ['name', 'year','semester', 'size'])
sta_663 = course(name = 'STA-663', year=2017, size=50, semester='Spring')
sta_663
course(name='STA-663', year=2017, semester='Spring', size=50)
name, *when, size = sta_663
when
[2017, 'Spring']
sta_663[-1]
50
sta_663.size
50
Lists¶
x = [1,2,3,4,5]
x[1:4]
[2, 3, 4]
x[-1] = 10
x
[1, 2, 3, 4, 10]
x[::2]
[1, 3, 10]
x[::-1]
[10, 4, 3, 2, 1]
x + x
[1, 2, 3, 4, 10, 1, 2, 3, 4, 10]
x * 3
[1, 2, 3, 4, 10, 1, 2, 3, 4, 10, 1, 2, 3, 4, 10]
x.append(20)
x
[1, 2, 3, 4, 10, 20]
x.extend([3,4,5])
x
[1, 2, 3, 4, 10, 20, 3, 4, 5]
x.index(10)
4
x.count(3)
2
Sets¶
s = {1,1,2,3,4}
s
{1, 2, 3, 4}
s.add(2)
s
{1, 2, 3, 4}
s.add(5)
s
{1, 2, 3, 4, 5}
Set operations and equivalent methods¶
s & {5,6,7}, s.intersection({5,6,7})
({5}, {5})
s | {5,6,7}, s.union({5,6,7})
({1, 2, 3, 4, 5, 6, 7}, {1, 2, 3, 4, 5, 6, 7})
s - {5,6,7}, s.difference({5,6,7})
({1, 2, 3, 4}, {1, 2, 3, 4})
s ^ {5,6,7}, s.symmetric_difference({5,6,7})
({1, 2, 3, 4, 6, 7}, {1, 2, 3, 4, 6, 7})
Dictionary¶
d = {'a': 1, 'b':2, 'c':3}
d
{'a': 1, 'b': 2, 'c': 3}
d['b']
2
d1 = dict(d=4, e=5, f=6)
d1
{'d': 4, 'e': 5, 'f': 6}
d.update(d1)
d
{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6}
list(d.keys())
['c', 'e', 'a', 'd', 'f', 'b']
list(d.values())
[3, 5, 1, 4, 6, 2]
d['g'] = 7
d
{'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6, 'g': 7}
for k in d:
print(k, d[k])
c 3
e 5
a 1
g 7
d 4
f 6
b 2
Dictionary variants¶
# From Python 3.6 regular dictionaries will maintain order
d = {}
d['z'] = 1
d['x'] = 2
d['y'] = 3
for k in d:
print(k, d[k])
x 2
y 3
z 1
d = collections.OrderedDict()
d['z'] = 1
d['x'] = 2
d['y'] = 3
for k in d:
print(k, d[k])
z 1
x 2
y 3
d = collections.defaultdict(list)
d['a'].append(1)
d['a'].append(2)
d['b'].extend([3,4,5])
d
defaultdict(list, {'a': [1, 2], 'b': [3, 4, 5]})
Example: Word counter¶
jabberwocky = '''
’Twas brillig, and the slithy toves
Did gyre and gimble in the wabe:
All mimsy were the borogoves,
And the mome raths outgrabe.
“Beware the Jabberwock, my son!
The jaws that bite, the claws that catch!
Beware the Jubjub bird, and shun
The frumious Bandersnatch!”
He took his vorpal sword in hand;
Long time the manxome foe he sought—
So rested he by the Tumtum tree
And stood awhile in thought.
And, as in uffish thought he stood,
The Jabberwock, with eyes of flame,
Came whiffling through the tulgey wood,
And burbled as it came!
One, two! One, two! And through and through
The vorpal blade went snicker-snack!
He left it dead, and with its head
He went galumphing back.
“And hast thou slain the Jabberwock?
Come to my arms, my beamish boy!
O frabjous day! Callooh! Callay!”
He chortled in his joy.
’Twas brillig, and the slithy toves
Did gyre and gimble in the wabe:
All mimsy were the borogoves,
And the mome raths outgrabe.
'''
Using regular dictionary¶
c1 = {}
for word in jabberwocky.split():
c1[word] = c1.get(word, 0) + 1
c1['vorpal']
2
Using defaultdict with int factory¶
int() # Note int is a factory fucntion that produces 0
0
c2 = collections.defaultdict(int)
for word in jabberwocky.split():
c2[word] += 1
c2['vorpal']
2
Control Structures¶
if-elif-else¶
x, y = 3,4
if (x > y):
print(x, '>', y)
elif (x == y):
print(x, 'equals', y)
else:
print('Either', x, '<', y, 'or x and y are not orderable')
Either 3 < 4 or x and y are not orderable
continue and break¶
for i in range(3):
for j in range(5):
if i==j:
continue
print(i, j)
0 1
0 2
0 3
0 4
1 0
1 2
1 3
1 4
2 0
2 1
2 3
2 4
i = 0
while True:
print(i)
if i > 5:
break
i += 1
0
1
2
3
4
5
6
Functions¶
Built-in functions¶
([x for x in dir(__builtin__) if x.islower() and not x.startswith('__')])
['abs',
'all',
'any',
'ascii',
'bin',
'bool',
'bytearray',
'bytes',
'callable',
'chr',
'classmethod',
'compile',
'complex',
'copyright',
'credits',
'delattr',
'dict',
'dir',
'divmod',
'dreload',
'enumerate',
'eval',
'exec',
'filter',
'float',
'format',
'frozenset',
'get_ipython',
'getattr',
'globals',
'hasattr',
'hash',
'help',
'hex',
'id',
'input',
'int',
'isinstance',
'issubclass',
'iter',
'len',
'license',
'list',
'locals',
'map',
'max',
'memoryview',
'min',
'next',
'object',
'oct',
'open',
'ord',
'pow',
'print',
'property',
'range',
'repr',
'reversed',
'round',
'set',
'setattr',
'slice',
'sorted',
'staticmethod',
'str',
'sum',
'super',
'tuple',
'type',
'vars',
'zip']
len('hello')
5
range(5, 10, 2)
range(5, 10, 2)
ord('c') - ord('a')
2
chr(ord('a') + 2)
'c'
list(zip('abcd', range(1,10)))
[('a', 1), ('b', 2), ('c', 3), ('d', 4)]
sum([4,5,6])
15
sorted(fruits)
['apple', 'banana', 'cherry', 'durian', 'eggplant', 'fig']
sorted(fruits, reverse=True)
['fig', 'eggplant', 'durian', 'cherry', 'banana', 'apple']
sorted(fruits, key=len)
['fig', 'apple', 'banana', 'cherry', 'durian', 'eggplant']
User-defined functions¶
def f(a, b, c):
return a + b * c
f(1,2,3)
7
f(c=3, a=1, b=2)
7
f(1,2,c=3)
7
args = [1,2,3]
f(*args)
7
kwargs = dict(a=1, b=2, c=3)
f(**kwargs)
7